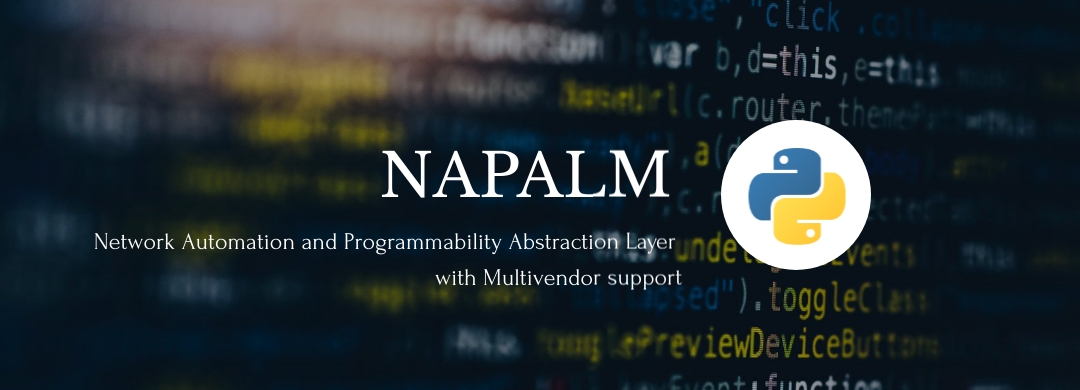
How to take your first steps in Network Automation with NAPALM? Cisco IOS and Cisco XR
Introduction
NAPALM (Network Automation and Programmability Abstraction Layer with Multivendor support) is a Python library that implements a set of functions to interact with different network device operating systems using a unified API.
NAPALM supports various methods to connect to devices, manipulate settings, or retrieve data.
The official team that maintains NAPALM, also known as the “Core Developers“, have made developments for multiple manufacturers and operating systems. They currently support:
- Arista EOS
- Cisco IOS
- Cisco IOS-XR
- Cisco NX-OS
- Juniper JunOS
In addition to the core drivers, napalm also supports community powered drivers. This community maintains its driver repositories on Github. Here you can find drivers for other manufacturers such as Huawei, Cumulus, HP, ArubaOS, among others.
In this tutorial we are going to take the first steps so that you can install NAPALM. We will continue to collect information from Cisco routers with the IOS and IOS-XR operating system and finally make configurations. Knowing these basic steps I am sure that the doors will be opened to dozens of ideas and use cases in which you can take advantage of this knowledge.
Knowledge Required for this Tutorial
To get the best out of this Tutorial, the following is required:
- Work environment is Ubuntu Linux or any distribution based on Ubuntu.
- Basic knowledge of Python is also required.
Step # 1 – Install Virtual Environment in Python
Recent versions of Ubuntu come with Python3 installed so you may not have to install it. To validate if you have Python3 installed on your Ubuntu, you can run this command:
python3 -V
The output should look like this:
Python 3.6.9
In case you don’t have Python 3 or the compatible version, you can install Python 3 from the Ubuntu repositories. If you don’t know how, you can see my tutorial on how to install Python 3.
In order not to permanently install NAPALM in our libraries, we will create a virtual working environment with Python. For that we will need to install the following package:
sudo apt-get install -y python3-venv
Once we have installed this package, we will create the virtual environment:
python3 -m venv virtual_env_napalm
To use this environment, you must activate it, which you can do by typing the following command that calls the activation script:
source virtual_env_napalm/bin/activate
Your Prompt will now be prefixed with the environment name, in this case it is called virtual_env_napalm. Your prefix may look somewhat different, but the name of your environment in parentheses should be the first thing you see on your command line, example:
(virtual_env_napalm) codingnetworks@lab-pc:~$
Step # 2 – Dependencies Installation
Now that we have our virtual environment mounted, we are going to install all the dependencies of NAPALM.
We must first get the Python PIP3 package manager. To install it you can run the following command:
sudo apt-get install python3-pip
Other dependencies that NAPALM has, specifically for IOS and IOS-XR operating systems are the following:
sudo apt-get install -y --force-yes libssl-dev libffi-dev python-dev python-cffi
We are now ready to install NAPALM.
Step # 3 – Installation of the NAPALM Library
The installation command is simple:
pip3 install napalm
Ready! Let’s immediately write our code.
Step # 4 – Connecting to a Cisco IOS and Cisco IOS-XR router
Our first task will be to write a code to connect to a Cisco router with IOS and another with IOS-XR. Below the code:
import napalm
def main():
# Use the appropriate network driver to connect to the device:
driver_ios = napalm.get_network_driver("ios")
driver_iosxr = napalm.get_network_driver("iosxr")
#Create routers objects
ios_router = driver_ios(
hostname="192.168.56.11",
username="codingnetworks",
password="codingnetworks",
optional_args={"inline_transfer": True}
)
iosxr_router = driver_iosxr(
hostname="192.168.56.31",
username="codingnetworks",
password="codingnetworks",
)
#Connect to the routers
print("Connecting to ios router ...")
ios_router.open()
print("Checking ios router Connection Status:")
print(ios_router.is_alive())
print("Connecting to IOS XR router ...")
iosxr_router.open()
print("Checking IOS XR router Connection Status:")
print(iosxr_router.is_alive())
# close the session with the device.
iosxr_router.close()
ios_router.close()
print("Done.")
if name == "main":
main()
Let’s take this code, save it to a file called “napalm_practice.py” and run it with the following command:
python3 napalm_practice.py
The output should look like this:
Connecting to ios router …
Checking ios router Connection Status:
{'is_alive': True}
Connecting to IOS XR router …
Checking IOS XR router Connection Status:
{'is_alive': True}
Done.
With this simple code we connect to two routers, validate the connection status and disconnect. We have just completed another step toward programmability and automation.
Step # 5 – Collect information from Devices
Let’s take the code from the previous step and add a few lines with which we are going to collect the ARP table from the two routers. Then this collected information will be presented on screen.
import napalm
import json
def main():
# Use the appropriate network driver to connect to the device:
driver_ios = napalm.get_network_driver("ios")
driver_iosxr = napalm.get_network_driver("iosxr")
#Create routers objects
ios_router = driver_ios(
hostname="192.168.56.11",
username="codingnetworks",
password="codingnetworks",
optional_args={"inline_transfer": True}
)
iosxr_router = driver_iosxr(
hostname="192.168.56.31",
username="codingnetworks",
password="codingnetworks",
)
#Connect to the routers
print("Connecting to ios router ...")
ios_router.open()
print("Connecting to IOS XR router ...")
iosxr_router.open()
#Getting ARP Table information
print("IOS XR router: Get ARP Table ...")
print(json.dumps(iosxr_router.get_arp_table(), indent=4))
print("IOS router: Get ARP Table ...")
print(json.dumps(ios_router.get_arp_table(), indent=4))
#Close the session with the device.
iosxr_router.close()
ios_router.close()
print("Done.")
if name == "main":
main()
Let’s take this code, save it to a file called “napalm_practice.py” and run it with the following command:
python3 napalm_practice.py
The output should look like this:
Connecting to ios router …
Checking ios router Connection Status:
{'is_alive': True}
Connecting to IOS XR router …
Checking IOS XR router Connection Status:
{'is_alive': True}
iosxr router: Get ARP Table …
[
{
"interface": "GigabitEthernet0/0/0/2",
"mac": "0A:00:27:00:00:16",
"ip": "192.168.56.1",
"age": 7.0
},
{
"interface": "GigabitEthernet0/0/0/2",
"mac": "50:00:00:0B:00:03",
"ip": "192.168.56.31",
"age": 0.0
}
]
ios router: Get ARP Table …
[
{
"interface": "GigabitEthernet0/1",
"mac": "50:00:00:06:00:01",
"ip": "172.17.10.1",
"age": 0.0
},
{
"interface": "GigabitEthernet0/3",
"mac": "0A:00:27:00:00:16",
"ip": "192.168.56.1",
"age": 0.0
},
{
"interface": "GigabitEthernet0/3",
"mac": "50:00:00:06:00:03",
"ip": "192.168.56.11",
"age": 0.0
}
]
Done.
Please note that this time we import the “json” module. The reason is that by converting a dictionary to JSON, it can be presented in a more “pretty” way.
Step # 6 – Perform Basic Configurations
It is time to do a basic configuration. What we will do is configure a description to a router interface with the IOS operating system.
import napalm
def main():
# Use the appropriate network driver to connect to the device:
driver_ios = napalm.get_network_driver("ios")
driver_iosxr = napalm.get_network_driver("iosxr")
#Create routers objects
ios_router = driver_ios(
hostname="192.168.56.11",
username="codingnetworks",
password="codingnetworks",
optional_args={"inline_transfer": True}
)
iosxr_router = driver_iosxr(
hostname="192.168.56.31",
username="codingnetworks",
password="codingnetworks",
)
#Connect to the routers
print("Connecting to ios router ...")
ios_router.open()
print("Connecting to IOS XR router ...")
iosxr_router.open()
#Loading ios router object with the needed configuration.
ios_router.load_merge_candidate(filename=None, config="interface gi0/2\ndescription Test")
print("\nDiff:")
print(ios_router.compare_config())
#Commit the configuration into the router.
print("Committing ...")
ios_router.commit_config()
# close the session with the device.
iosxr_router.close()
ios_router.close()
print("Done.")
if name == "main":
main()
Let’s take this code, save it to a file called “napalm_practice.py” and run it with the following command:
python3 napalm_practice.py
The output should look like this:
Connecting to ios router …
Checking ios router Connection Status:
{'is_alive': True}
Connecting to IOS XR router …
Checking IOS XR router Connection Status:
{'is_alive': True}
Diff:
+interface gi0/2
+description Test
Committing …
Done.
Continue learning
I hope this code has served as a basis for you to get started with the NAPALM module and your adventures with Programming and Network Automation. Here are several links where you can expand your knowledge about NAPALM:
NAPALM Official Documentation:
https://napalm.readthedocs.io/en/latest/
NAPALM supported methods to collect information or modify configuration in equipment.
https://napalm.readthedocs.io/en/latest/support/index.html
Details of each method, parameters, what they return, can be found at the following link:
https://napalm.readthedocs.io/en/latest/base.html
If you have questions or doubts about what you saw in this post or about what you find in the official documentation, comment below and we will gladly clarify your doubts.