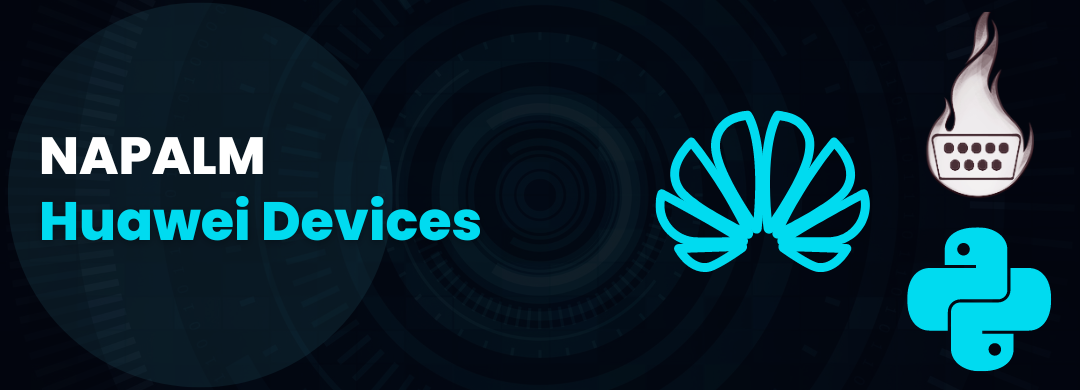
NAPALM Network Automation Python: Working with Huawei VRP
Introduction
In this article we are going to learn the functions and methods of NAPALM to collect data on Huawei VRP routers. We will write multiple Python scripts to collect data from a network, which you can use to create reports or investigate a problem. This is a continuation of the article in which we work with Cisco IOS and IOS-XR and it is part of the adventure in which I want to show you how you can implement Network Programmability and Automation with Python and the NAPALM library.
The main content is in the following video. So start here so that you can understand some sections of the article.
NAPALM Plugin: Huawei-CE
As you will remember on the official page, this VRP Operating System is not listed as one of the OS supported by NAPALM. This particular case is a community development.
Community developments are known as NAPALM Plugins because they do not come in the official library and are not maintained by Core Developers. But without they are hosted on a common page created by them, called the NAPALM Automation Community.
To see this list of Plugins, we first go to the “napalm.readthedocs.io” page, section Contribuiting, Proposing a new driver, then Community Driver and finally here we will find a link that will take us to the page where the collection of developed plugins are.
The plugin that we are going to use to work with Huawei devices is called napalm-ce. Here are the methods supported by this plugin:
cli(commands)
close()
commit_config()
compare_config()
discard_config()
get_arp_table()
get_config(retrieve=u'all')
get_environment()
get_facts()
get_interfaces()
get_interfaces_counters()
get_interfaces_ip()
get_lldp_neighbors()
get_mac_address_table()
get_users()
is_alive()
load_merge_candidate(filename=None, config=None)
load_replace_candidate(filename=None, config=None)
open()
ping(destination, source=u'', ttl=255, timeout=2, size=100, count=5, vrf=u'')
rollback()
Installing the NAPALM-CE Plugin
To install napalm-ce you just have to run the following command:
pip install napalm-ce
NAPALM CLI
As we already know, the NAPALM installation comes with a tool that allows us to use NAPALM directly from the command line. Its use is quite simple and we can see how it is used in the command’s help menu.
From this tool it is possible to execute configuration operations on the devices, validate configurations or states and make calls to the NAPALM methods to obtain data from the devices.
This time we are going to use this tool to test the Huawei-CE Plugin on one of the network switches that we saw in the video.
Let’s see an example:
napalm --user codingnetworks --password Coding.Networks1 --vendor ce VRP-SW2 call get_interfaces
Excerpt from Output:
"Vlanif1": {
"description": " ",
"is_enabled": true,
"is_up": true,
"last_flapped": -1.0,
"mac_address": "70:7B:E8:66:35:99",
"duplex": "",
"speed": -1,
"mtu": "1500",
"port_bw": "",
"rx_optic": "",
"tx_optic": "",
"crc_errors": ""
},
"Vlanif10": {
"description": " ",
"is_enabled": true,
"is_up": true,
"last_flapped": -1.0,
"mac_address": "70:7B:E8:66:35:99",
"duplex": "",
"speed": -1,
"mtu": "1500",
"port_bw": "",
"rx_optic": "",
"tx_optic": "",
"crc_errors": ""
}
NAPALM Python
It is in this section where we will begin to see and enjoy the benefits of using NAPALM for programmability and network automation in Huawei devices.
NAPALM Python Information and Interface Table
We are going to modify the script that we did in the video Working with Cisco IOS and IOS-XR so that it prints information such as the hostname, manufacturer, model, time they have in operation and the serial number of Huawei devices in a table. Here is the modified code:
import napalm from tabulate import tabulate def main(): driver_vrp = napalm.get_network_driver("ce") device_list = [["vrp-sw2","vrp", "switch"],["vrp-r2", "vrp", "router"], ["vrp-r3", "vrp", "router"],["vrp-r1", "vrp", "router"],["vrp-sw1", "vrp", "switch"]] network_devices = [] for device in device_list: network_devices.append( driver_vrp( hostname = device[0], username = "codingnetworks", password = "Coding.Networks1" ) ) devices_table = [["hostname", "vendor", "model", "uptime", "serial_number"]] devices_table_int = [["hostname","interface","is_up", "is_enabled", "description", "speed", "mtu", "mac_address"]] for device in network_devices: print("Connecting to {} ...".format(device.hostname)) device.open() print("Getting device facts") device_facts = device.get_facts() devices_table.append([device_facts["hostname"], device_facts["vendor"], device_facts["model"], device_facts["uptime"], device_facts["serial_number"] ]) print("Getting device interfaces") device_interfaces = device.get_interfaces() for interface in device_interfaces: devices_table_int.append([device_facts["hostname"], interface, device_interfaces[interface]['is_up'], device_interfaces[interface]['is_enabled'], device_interfaces[interface]['description'], device_interfaces[interface]['speed'], device_interfaces[interface]['mtu'], device_interfaces[interface]['mac_address'] ]) device.close() print("Done.") print(tabulate(devices_table, headers="firstrow")) print() print(tabulate(devices_table_int, headers="firstrow")) if __name__ == '__main__': main()
In the video you will find the explanation of this code.
Let’s see the output of the Script:
Connecting to vrp-sw2 ...
Getting device facts
Getting device interfaces
Done.
Connecting to vrp-r2 ...
Getting device facts
Getting device interfaces
Done.
Connecting to vrp-r3 ...
Getting device facts
Getting device interfaces
Done.
Connecting to vrp-r1 ...
Getting device facts
Getting device interfaces
Done.
Connecting to vrp-sw1 ...
Getting device facts
Getting device interfaces
Done.
hostname vendor model uptime serial_number
---------- -------- ------- -------- ---------------
VRP-SW2 Huawei CE6800 240 []
VRP-R2 Huawei NE40E 240 []
VRP-R3 Huawei NE40E 240 []
VRP-R1 Huawei NE40E 240 []
VRP-SW1 Huawei CE6800 300 []
hostname interface is_up is_enabled description speed mtu mac_address
---------- -------------------- ------- ------------ ----------------------------- ------- ----- -----------------
VRP-SW2 GE1/0/0 True True To Cloud Management -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/1 True True to_VRP-R1 Eth 1/0/1 - LAN -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/2 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/3 True True to_PC1 - LAN -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/4 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/5 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/6 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/7 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/8 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/9 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/10 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/11 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/12 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/13 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/14 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/15 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/16 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/17 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/18 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/19 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/20 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/21 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/22 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/23 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/24 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/25 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/26 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/27 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/28 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/29 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/30 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/31 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/32 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/33 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/34 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/35 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/36 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/37 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/38 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/39 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/40 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/41 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/42 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/43 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/44 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/45 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/46 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/47 False False -1 70:7B:E8:66:35:99
VRP-SW2 MEth0/0/0 False True -1 1500 70:7B:E8:66:35:98
VRP-SW2 NULL0 True True -1 1500
VRP-SW2 Vlanif1 True True -1 1500 70:7B:E8:66:35:99
VRP-SW2 Vlanif10 True True -1 1500 70:7B:E8:66:35:99
VRP-R2 Ethernet1/0/0 False True to_VRP-SW2 GE 1/0/1 - LAN -1 1500 38:1F:01:01:01:00
VRP-R2 Ethernet1/0/0.30 True True to_VRP-SW1 GE 1/0/0 - VLAN 30 -1 1500 38:1F:01:01:01:00
VRP-R2 Ethernet1/0/1 False True -1 1500 38:1F:01:01:01:01
VRP-R2 Ethernet1/0/2 True True to_VRP-R1 Eth 1/0/2 - LAN -1 1500 38:1F:01:01:01:02
VRP-R2 Ethernet1/0/3 False True -1 1500 38:1F:01:01:01:03
VRP-R2 Ethernet1/0/4 False True -1 1500 38:1F:01:01:01:04
VRP-R2 Ethernet1/0/5 False True -1 1500 38:1F:01:01:01:05
VRP-R2 Ethernet1/0/6 False True -1 1500 38:1F:01:01:01:06
VRP-R2 Ethernet1/0/7 False True -1 1500 38:1F:01:01:01:07
VRP-R2 Ethernet1/0/8 False True -1 1500 38:1F:01:01:01:08
VRP-R2 Ethernet1/0/9 False True -1 1500 38:1F:01:01:01:09
VRP-R2 GigabitEthernet0/0/0 False True -1 1500 38:1F:01:01:11:10
VRP-R2 LoopBack0 True True to_VRP-SW2 GE 1/0/1 - VLAN 1 -1 1500
VRP-R2 NULL0 True True -1 1500
VRP-R3 Ethernet1/0/0 True True to_VRP-R1 GE 1/0/0 - LAN -1 1500 38:1F:01:03:01:00
VRP-R3 Ethernet1/0/1 False True -1 1500 38:1F:01:03:01:01
VRP-R3 Ethernet1/0/2 False True -1 1500 38:1F:01:03:01:02
VRP-R3 Ethernet1/0/3 False True -1 1500 38:1F:01:03:01:03
VRP-R3 Ethernet1/0/4 False True -1 1500 38:1F:01:03:01:04
VRP-R3 Ethernet1/0/5 False True -1 1500 38:1F:01:03:01:05
VRP-R3 Ethernet1/0/6 False True -1 1500 38:1F:01:03:01:06
VRP-R3 Ethernet1/0/7 False True -1 1500 38:1F:01:03:01:07
VRP-R3 Ethernet1/0/8 False True -1 1500 38:1F:01:03:01:08
VRP-R3 Ethernet1/0/9 False True -1 1500 38:1F:01:03:01:09
VRP-R3 GigabitEthernet0/0/0 False True -1 1500 38:1F:01:03:11:10
VRP-R3 LoopBack0 True True Management IP -1 1500
VRP-R3 LoopBack1 True True Management IP -1 1500
VRP-R3 NULL0 True True -1 1500
VRP-R1 Ethernet1/0/0 True True to_VRP-R2 Eth 1/0/0 - LAN -1 1500 38:1F:01:02:01:00
VRP-R1 Ethernet1/0/1 False True to_VRP-SW2 GE 1/0/1 - LAN -1 1500 38:1F:01:02:01:01
VRP-R1 Ethernet1/0/1.1 True True to_VRP-SW2 GE 1/0/1 - VLAN 1 -1 1500 38:1F:01:02:01:01
VRP-R1 Ethernet1/0/1.10 True True to_VRP-SW2 GE 1/0/1 - VLAN 10 -1 1500 38:1F:01:02:01:01
VRP-R1 Ethernet1/0/2 True True to_VRP-R3 Eth 1/0/2 - LAN -1 1500 38:1F:01:02:01:02
VRP-R1 Ethernet1/0/3 False True -1 1500 38:1F:01:02:01:03
VRP-R1 Ethernet1/0/4 False True -1 1500 38:1F:01:02:01:04
VRP-R1 Ethernet1/0/5 False True -1 1500 38:1F:01:02:01:05
VRP-R1 Ethernet1/0/6 False True -1 1500 38:1F:01:02:01:06
VRP-R1 Ethernet1/0/7 False True -1 1500 38:1F:01:02:01:07
VRP-R1 Ethernet1/0/8 False True -1 1500 38:1F:01:02:01:08
VRP-R1 Ethernet1/0/9 False True -1 1500 38:1F:01:02:01:09
VRP-R1 GigabitEthernet0/0/0 False True -1 1500 38:1F:01:02:11:10
VRP-R1 LoopBack0 True True to_VRP-SW2 GE 1/0/1 - VLAN 1 -1 1500
VRP-R1 NULL0 True True -1 1500
VRP-SW1 GE1/0/0 True True to_VRP-R2 Eth 1/0/0 - LAN -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/1 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/2 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/3 True True to_PC1 - LAN -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/4 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/5 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/6 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/7 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/8 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/9 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/10 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/11 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/12 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/13 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/14 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/15 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/16 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/17 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/18 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/19 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/20 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/21 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/22 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/23 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/24 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/25 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/26 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/27 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/28 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/29 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/30 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/31 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/32 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/33 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/34 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/35 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/36 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/37 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/38 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/39 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/40 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/41 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/42 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/43 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/44 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/45 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/46 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/47 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 MEth0/0/0 False True -1 1500 70:7B:E8:B0:04:A4
VRP-SW1 NULL0 True True -1 1500
VRP-SW1 Vlanif30 True True -1 1500 70:7B:E8:B0:04:A5
NAPALM Python Mac Address Table
We are going to modify the code above to add other functionality. This functionality will allow the script to print a table with the mac addresses table of the switches. Below the code:
import napalm from tabulate import tabulate def main(): driver_vrp = napalm.get_network_driver("ce") device_list = [["vrp-sw2","vrp", "switch"],["vrp-r2", "vrp", "router"], ["vrp-r3", "vrp", "router"],["vrp-r1", "vrp", "router"],["vrp-sw1", "vrp", "switch"]] network_devices = [] for device in device_list: network_devices.append( driver_vrp( hostname = device[0], username = "codingnetworks", password = "Coding.Networks1" ) ) devices_table = [["hostname", "vendor", "model", "uptime", "serial_number"]] devices_table_int = [["hostname","interface","is_up", "is_enabled", "description", "speed", "mtu", "mac_address"]] devices_mac_table = [["hostname","mac", "ïnterface", "vlan", "static"]] for device in network_devices: print("Connecting to {} ...".format(device.hostname)) device.open() print("Getting device facts") device_facts = device.get_facts() devices_table.append([device_facts["hostname"], device_facts["vendor"], device_facts["model"], device_facts["uptime"], device_facts["serial_number"] ]) print("Getting device interfaces") device_interfaces = device.get_interfaces() for interface in device_interfaces: devices_table_int.append([device_facts["hostname"], interface, device_interfaces[interface]['is_up'], device_interfaces[interface]['is_enabled'], device_interfaces[interface]['description'], device_interfaces[interface]['speed'], device_interfaces[interface]['mtu'], device_interfaces[interface]['mac_address'] ]) if "SW" in device_facts["hostname"]: print("Getting Mac Address Table from Switch") device_mac_info = device.get_mac_address_table() for mac_entry in device_mac_info: devices_mac_table.append([device_facts["hostname"], mac_entry["mac"], mac_entry["interface"], mac_entry["vlan"], mac_entry["static"], ]) device.close() print("Done.") print(tabulate(devices_table, headers="firstrow")) print() print(tabulate(devices_table_int, headers="firstrow")) print() print(tabulate(devices_mac_table, headers="firstrow")) if __name__ == '__main__': main()
In the video you will find the explanation of this code.
Let’s see the output of this code:
Connecting to vrp-sw2 ...
Getting device facts
Getting device interfaces
Getting Mac Address Table from Switch
Done.
Connecting to vrp-r2 ...
Getting device facts
Getting device interfaces
Done.
Connecting to vrp-r3 ...
Getting device facts
Getting device interfaces
Done.
Connecting to vrp-r1 ...
Getting device facts
Getting device interfaces
Done.
Connecting to vrp-sw1 ...
Getting device facts
Getting device interfaces
Getting Mac Address Table from Switch
Done.
hostname vendor model uptime serial_number
---------- -------- ------- -------- ---------------
VRP-SW2 Huawei CE6800 240 []
VRP-R2 Huawei NE40E 240 []
VRP-R3 Huawei NE40E 240 []
VRP-R1 Huawei NE40E 240 []
VRP-SW1 Huawei CE6800 300 []
hostname interface is_up is_enabled description speed mtu mac_address
---------- -------------------- ------- ------------ ----------------------------- ------- ----- -----------------
VRP-SW2 GE1/0/0 True True To Cloud Management -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/1 True True to_VRP-R1 Eth 1/0/1 - LAN -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/2 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/3 True True to_PC1 - LAN -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/4 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/5 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/6 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/7 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/8 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/9 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/10 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/11 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/12 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/13 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/14 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/15 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/16 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/17 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/18 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/19 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/20 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/21 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/22 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/23 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/24 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/25 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/26 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/27 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/28 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/29 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/30 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/31 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/32 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/33 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/34 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/35 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/36 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/37 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/38 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/39 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/40 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/41 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/42 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/43 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/44 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/45 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/46 False False -1 70:7B:E8:66:35:99
VRP-SW2 GE1/0/47 False False -1 70:7B:E8:66:35:99
VRP-SW2 MEth0/0/0 False True -1 1500 70:7B:E8:66:35:98
VRP-SW2 NULL0 True True -1 1500
VRP-SW2 Vlanif1 True True -1 1500 70:7B:E8:66:35:99
VRP-SW2 Vlanif10 True True -1 1500 70:7B:E8:66:35:99
VRP-R2 Ethernet1/0/0 False True to_VRP-SW2 GE 1/0/1 - LAN -1 1500 38:1F:01:01:01:00
VRP-R2 Ethernet1/0/0.30 True True to_VRP-SW1 GE 1/0/0 - VLAN 30 -1 1500 38:1F:01:01:01:00
VRP-R2 Ethernet1/0/1 False True -1 1500 38:1F:01:01:01:01
VRP-R2 Ethernet1/0/2 True True to_VRP-R1 Eth 1/0/2 - LAN -1 1500 38:1F:01:01:01:02
VRP-R2 Ethernet1/0/3 False True -1 1500 38:1F:01:01:01:03
VRP-R2 Ethernet1/0/4 False True -1 1500 38:1F:01:01:01:04
VRP-R2 Ethernet1/0/5 False True -1 1500 38:1F:01:01:01:05
VRP-R2 Ethernet1/0/6 False True -1 1500 38:1F:01:01:01:06
VRP-R2 Ethernet1/0/7 False True -1 1500 38:1F:01:01:01:07
VRP-R2 Ethernet1/0/8 False True -1 1500 38:1F:01:01:01:08
VRP-R2 Ethernet1/0/9 False True -1 1500 38:1F:01:01:01:09
VRP-R2 GigabitEthernet0/0/0 False True -1 1500 38:1F:01:01:11:10
VRP-R2 LoopBack0 True True to_VRP-SW2 GE 1/0/1 - VLAN 1 -1 1500
VRP-R2 NULL0 True True -1 1500
VRP-R3 Ethernet1/0/0 True True to_VRP-R1 GE 1/0/0 - LAN -1 1500 38:1F:01:03:01:00
VRP-R3 Ethernet1/0/1 False True -1 1500 38:1F:01:03:01:01
VRP-R3 Ethernet1/0/2 False True -1 1500 38:1F:01:03:01:02
VRP-R3 Ethernet1/0/3 False True -1 1500 38:1F:01:03:01:03
VRP-R3 Ethernet1/0/4 False True -1 1500 38:1F:01:03:01:04
VRP-R3 Ethernet1/0/5 False True -1 1500 38:1F:01:03:01:05
VRP-R3 Ethernet1/0/6 False True -1 1500 38:1F:01:03:01:06
VRP-R3 Ethernet1/0/7 False True -1 1500 38:1F:01:03:01:07
VRP-R3 Ethernet1/0/8 False True -1 1500 38:1F:01:03:01:08
VRP-R3 Ethernet1/0/9 False True -1 1500 38:1F:01:03:01:09
VRP-R3 GigabitEthernet0/0/0 False True -1 1500 38:1F:01:03:11:10
VRP-R3 LoopBack0 True True Management IP -1 1500
VRP-R3 LoopBack1 True True Management IP -1 1500
VRP-R3 NULL0 True True -1 1500
VRP-R1 Ethernet1/0/0 True True to_VRP-R2 Eth 1/0/0 - LAN -1 1500 38:1F:01:02:01:00
VRP-R1 Ethernet1/0/1 False True to_VRP-SW2 GE 1/0/1 - LAN -1 1500 38:1F:01:02:01:01
VRP-R1 Ethernet1/0/1.1 True True to_VRP-SW2 GE 1/0/1 - VLAN 1 -1 1500 38:1F:01:02:01:01
VRP-R1 Ethernet1/0/1.10 True True to_VRP-SW2 GE 1/0/1 - VLAN 10 -1 1500 38:1F:01:02:01:01
VRP-R1 Ethernet1/0/2 True True to_VRP-R3 Eth 1/0/2 - LAN -1 1500 38:1F:01:02:01:02
VRP-R1 Ethernet1/0/3 False True -1 1500 38:1F:01:02:01:03
VRP-R1 Ethernet1/0/4 False True -1 1500 38:1F:01:02:01:04
VRP-R1 Ethernet1/0/5 False True -1 1500 38:1F:01:02:01:05
VRP-R1 Ethernet1/0/6 False True -1 1500 38:1F:01:02:01:06
VRP-R1 Ethernet1/0/7 False True -1 1500 38:1F:01:02:01:07
VRP-R1 Ethernet1/0/8 False True -1 1500 38:1F:01:02:01:08
VRP-R1 Ethernet1/0/9 False True -1 1500 38:1F:01:02:01:09
VRP-R1 GigabitEthernet0/0/0 False True -1 1500 38:1F:01:02:11:10
VRP-R1 LoopBack0 True True to_VRP-SW2 GE 1/0/1 - VLAN 1 -1 1500
VRP-R1 NULL0 True True -1 1500
VRP-SW1 GE1/0/0 True True to_VRP-R2 Eth 1/0/0 - LAN -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/1 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/2 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/3 True True to_PC1 - LAN -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/4 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/5 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/6 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/7 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/8 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/9 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/10 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/11 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/12 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/13 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/14 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/15 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/16 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/17 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/18 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/19 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/20 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/21 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/22 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/23 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/24 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/25 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/26 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/27 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/28 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/29 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/30 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/31 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/32 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/33 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/34 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/35 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/36 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/37 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/38 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/39 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/40 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/41 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/42 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/43 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/44 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/45 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/46 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 GE1/0/47 False False -1 70:7B:E8:B0:04:A5
VRP-SW1 MEth0/0/0 False True -1 1500 70:7B:E8:B0:04:A4
VRP-SW1 NULL0 True True -1 1500
VRP-SW1 Vlanif30 True True -1 1500 70:7B:E8:B0:04:A5
hostname mac ïnterface vlan static
---------- ----------------- ----------- ------ --------
VRP-SW2 0A:00:27:00:00:19 GE1/0/0 1 False
VRP-SW2 38:1F:01:02:01:01 GE1/0/1 1 False
VRP-SW2 38:1F:01:02:01:01 GE1/0/1 10 False
VRP-SW1 38:1F:01:01:01:00 GE1/0/0 30 False
Conclusion
Isn’t all this surprising? We have seen again how with so few codes we can obtain so much information from a network and thus present it in a table, summarized, easy to read and understand. With those few functions you can collect data of your entire network and see what is connected to all your switches and routers.
If there is a problem in your network you can quickly see if there are Down interfaces that should not be Down, see if the switches stopped learning any MAC Addresses.
The time it takes to connect via CLI to all these devices to obtain this information and interpret it is reduced by more than 100% when you do it by applying Network Programmability.
Hello Michael,
First of all congrats for your great work both on your website and your youtube channel as well.
In my work network we use bellow vrp routers …
dis version
Huawei Versatile Routing Platform Software
VRP (R) software, Version 5.170 (AR2200 V200R009C00SPC500)
Copyright (C) 2011-2018 HUAWEI TECH CO., LTD
Huawei AR2204-27GE
As you can see the sysname of the router is NAME-ES-AR2204.
When i run your script i get below error …
netmiko.exceptions.ReadTimeout:
Pattern not detected: ” in output.
Backslashes are added inth routers sysname.
I generally have ssh access on the router and am able to run all APIs (get facts, get interfaces e.t.c)
I googled it and found that in some cases if we use the optional argument ‘global_delay_factor’: 2 or 4 the problem is solved.
But not in my case.
Do you have any idea how to solve this and why this is happening ?
Kind Regards,
Hi Chris, thank you and sorry for the big late response. In my video, i didn’t mention it but i had all router’s name configured in the local DNS(Host file), thats why i could use the names in my script. Maybe that’s the reason is not working for you. Use IP addreses and let me know how it goes.